DIY programming for your Microsoft Office products
The VBA programming platform that runs throughout nearly all Microsoft Office products is one of the most powerful tools anyone can use to enhance their use of those products.
This VBA guide for beginners will show you how to add the Developer menu to your Office application, how to enter the VBA editor window, and how basic VBA statements and loops work so that you can get started using VBA in Excel, Word, Powerpoint, Outlook, and OneNote.
This VBA guide uses the latest version of Microsoft Office products. If you have an earlier version you may see some slight differences from the screenshots.
How To Enable & Use The VBA Editor
In any of the Office products used in this guide, you may notice that you don’t have the referenced Developer menu. The developer menu is only available in Excel, Word, Outlook, and Powerpoint. OneNote doesn’t offer a tool to edit VBA code from inside the application, but you can still reference the OneNote API to interact with OneNote from other Office programs.
You’ll learn how to do this in our upcoming Advanced VBA guide.
- To enable the Developer menu in any office product, select the File menu, and select Options from the left navigation menu.
- You’ll see an Options menu pop-up. Select Customize Ribbon from the left navigation menu.
The left list includes all available menus and menu commands available in that Office application. The list on the right are those that are currently available or activated.
- You should see Developer in the list on the right, but it will not be activated. Just select the checkbox to activate the Developer menu.
- If you don’t see Developer available on the right, then change the left Choose commands from dropdown to All Commands. Find Developer from the list and select Add>> in the center to add that menu to the Ribbon.
- Select OK when you’re done.
- Once the Developer menu is active, you can go back to your main application window and select Developer from the top menu.
- Then select View Code from the Controls group in the ribbon to open the VBA editor window.
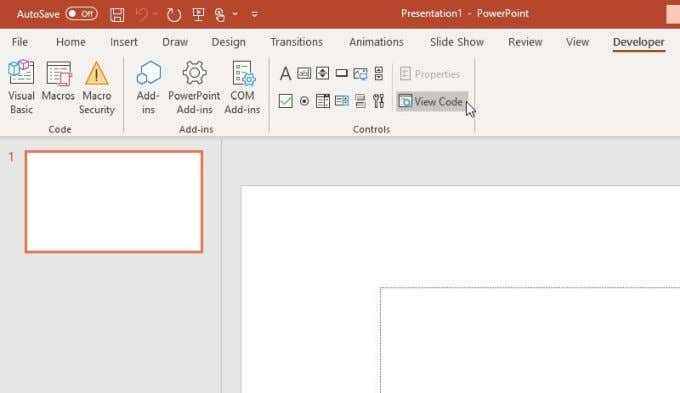
- This will open the VBA editor window where you can type the code you’ll learn in the next few sections.
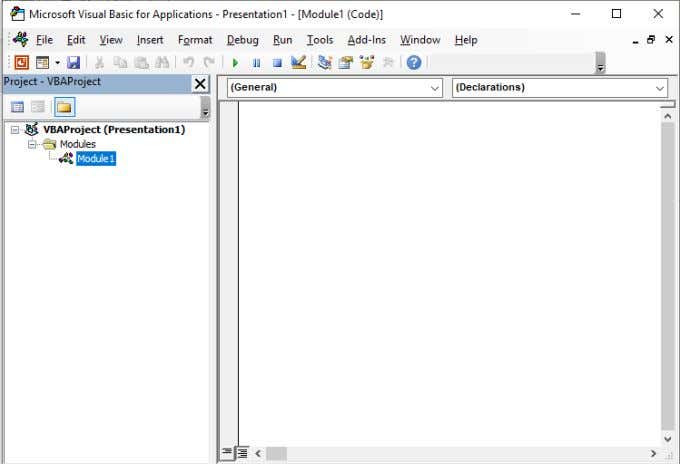
- Try adding the Developer menu to a few of the Office applications you use every day. Once you’re comfortable opening the VBA editor window, continue on to the next section of this guide.
General VBA Programming Tips for Beginners
You’ll notice when the VBA editor opens, the navigation options in the left panel look different from one Office application to the other.
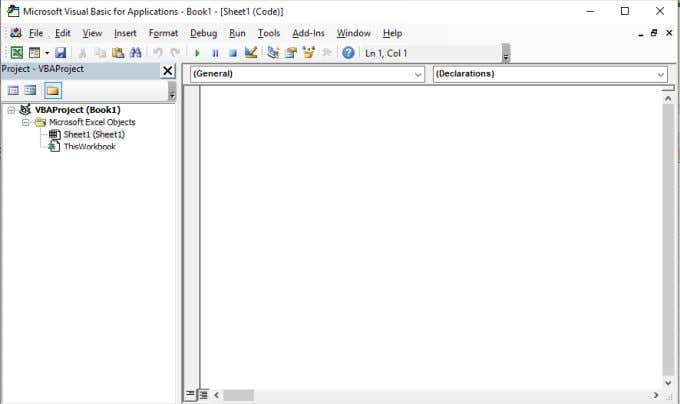
This is because the available objects where you can place VBA code depend on what objects there are in the application. For example, in Excel, you can add VBA code to workbook or sheet objects. In Word, you can add VBA code to documents. In Powerpoint, only to modules.
So, don’t be surprised by the different menus. The structure and syntax of the VBA code is the same across all applications. The only difference are the objects you can reference and the actions you can take on those objects through the VBA code.
Before we dive into the different objects and actions you can take on them via VBA code, let’s first look at the most common VBA structure and syntax you can use when you write VBA code.
Where To Put VBA Code
When you’re in the VBA editor, you need to use the two dropdown boxes at the top of the editing window to choose which object you want to attach the code to, and when you want the code to run.
For example, in Excel, if you choose Worksheet and Activate, the code will run whenever the worksheet is opened.
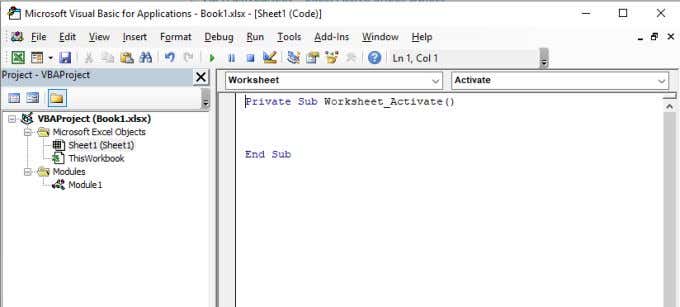
Other worksheet actions you can use to trigger your VBA code include when the worksheet changes, when it’s closed (deactivated), when the worksheet calculation is run, and more.
When you add VBA code in the editor, always make sure to place your VBA code on the object and using the correct action that you want to use to trigger that code.
VBA IF Statements
An IF statement works in VBA just like it works in any other programming language.
The first part of the IF statement looks at whether a condition or set of conditions is true. These conditions can be joined by an AND or OR operator to link them together.
One example would be to check if a grade in a spreadsheet is above or below a “passing” grade, and assigning the pass or fail status to another cell.
If Cells(2, 2) > 75 Then Cells(2, 3) = “Pass” Else Cells(2, 3) = “Fail”
If you don’t want the entire statement on a single line, you can split it into multiple lines by adding a “_” symbol at the end of the lines.
If Cells(2, 2) > 75 Then _
Cells(2, 3) = “Pass” Else _
Cells(2, 3) = “Fail”
Using this technique can often make code much easier to read and debug.
VBA For Next Loops
IF statements are great for single comparisons, like the example above of looking at a single cell. But what if you want to loop through an entire range of cells and do the same IF statement on each?
In this case you’d need a FOR loop.
To do this, you’d need to use the length of a range, and loop through that length by the number of rows that contain data.
To do this, you need to define the range and cell variables, and loop through them. You’ll also need to define a counter so you can output the results to the appropriate row. So your VBA code would first have this line.
Dim rng As Range, cell As Range
Dim rowCounter as Integer
Define the range size as follows.
Set rng = Range(“B2:B7”)
rowCounter = 2
Finally, you can create your FOR loop to step through every cell in that range and do the comparison.
For Each cell In rng If cell.Value > 75 Then _ Cells(rowCounter, 3) = "Pass" Else _ Cells(rowCounter, 3) = "Fail" rowCounter = rowCounter + 1 Next cell
Once this VBA script is run, you see the results in the actual spreadsheet.
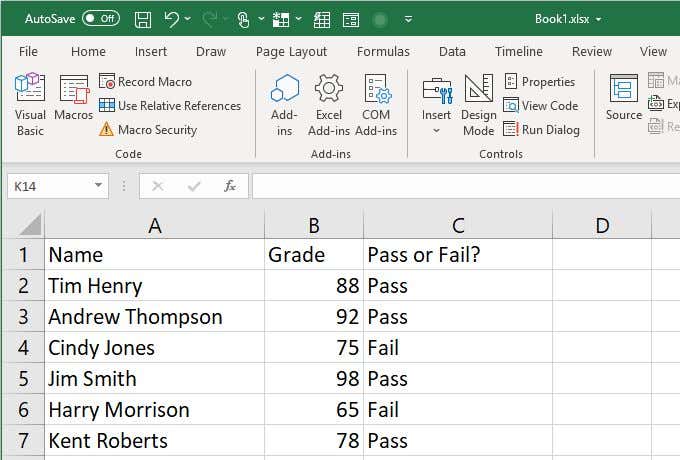
VBA While Loops
A While Loop also loops through a series of statements, just like the FOR loop, but the condition of the looping to continue is a condition remaining true.
For example, you could write the same FOR loop above, as a WHILE loop, by simply using the rowCounter variable as follows.
While rowCounter < rng.Count + 2 If Cells(rowCounter, 2) > 75 Then _ Cells(rowCounter, 3) = "Pass" Else _ Cells(rowCounter, 3) = "Fail" rowCounter = rowCounter + 1 Wend
Note: the rng.Count + 2 terminating limit is required because the row counter starts at 2 and needs to end on row 7 where the data ends. However, the count of the range (B2:B7) is only 6, and the While loop will only end once the counter is GREATER than the counter – so the last rowCounter value needs to be 8 (or rng.Count + 2).
You could also set up the While loop as follows:
While rowCounter <= rng.Count + 1
You can only increment the range count (6) by 1, because once the rowCounter variable reaches the end of the data (row 7), the loop can finish.
VBA Do While and Do Until Loops
Do While and Do Until loops are nearly identical to While loops, but work slightly different.
- The While Loop checks whether a condition is true at the beginning of the loop.
- The Do-While Loop checks whether a condition is true after executing the statements in the loop.
- The Do-Until Loop checks whether a condition is still false after executing the loop.
In this case you would rewrite the While loop above as follows, as a Do-While loop.
Do If Cells(rowCounter, 2) > 75 Then _ Cells(rowCounter, 3) = "Pass" Else _ Cells(rowCounter, 3) = "Fail" rowCounter = rowCounter + 1 Loop While rowCounter < rng.Count + 2
In this case the logic doesn’t change very much, but if you want to make sure the logic comparison takes place after all of the statements are run (allowing them all to run no matter what at least once), then a Do-While or Do-Until loop is the right option.
VBA Select Case Statements
The final type of logical statement you’ll need to understand to start structuring your VBA code are Select Case statements.
Given the example above, let’s say you want to have a grading method that isn’t just pass fail. Instead, you want to assign a letter grade from A through F.
You could do this with the following Select Case statement:
For Each cell In rng Select Case cell Case 95 To 100 Cells(rowCounter, 3) = "A" Case 85 To 94 Cells(rowCounter, 3) = "B" Case 75 To 84 Cells(rowCounter, 3) = "C" Case 65 To 74 Cells(rowCounter, 3) = "D" Case 0 To 64 Cells(rowCounter, 3) = "F" End Select rowCounter = rowCounter + 1 Next cell
The resulting spreadsheet after this VBA script runs looks like the one below.
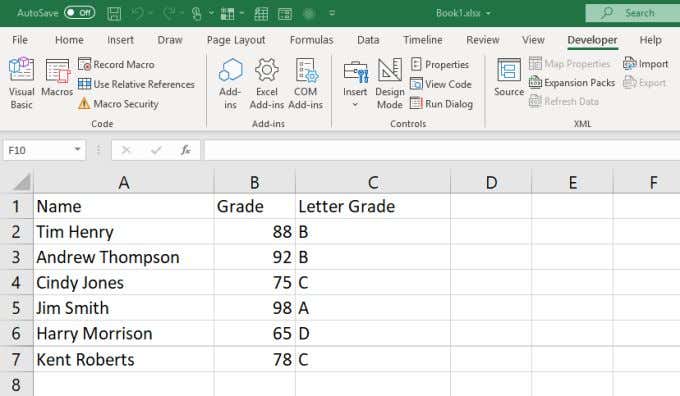
Now you know everything you need to know to start using VBA in your Microsoft Office applications.