Extremely useful and only limited by your imagination
VBA has been part of the Microsoft Office suite for many years. While it doesn’t have the full functionality and power of a full VB application, VBA provides Office users with the flexibility to integrate Office products and automate the work you do in them.
One of the most powerful tools available in VBA is the ability to load an entire range of data into a single variable called an array. By loading data in this way, you can manipulate or perform calculations on that range of data in a variety of ways.
So what is a VBA array? In this article we’ll answer that question, and show you how to use one in your own VBA script.
What Is a VBA Array?
Using a VBA array in Excel is very simple, but understanding the concept of arrays can be a little bit complex if you’ve never used one.
Think of an array like a box with sections inside it. A one-dimensional array is a box with one line of sections. A two-dimensional array is a box with two lines of sections.
You can put data into each section of this “box” in any order you like.
At the beginning of your VBA script, you need to define this “box” by defining your VBA array. So to create an array that can hold one set of data (a one-dimension array), you would write the following line.
Dim arrMyArray(1 To 6) As String
Later in your program, you can place data into any section of this array by referencing the section inside of parentheses.
arrMyArray(1) = "Ryan Dube"
You can create a two-dimensional array using the following line.
Dim arrMyArray(1 To 6,1 to 2) As Integer
The first number represents the row, and the second is the column. So the above array could hold a range with 6 rows and 2 columns.
You can load any element of this array with data as follows.
arrMyArray(1,2) = 3
This will load a 3 into the cell B1.
An array can hold any type of data as a regular variable, such as strings, boolean, integers, floats and more.
The number inside the parenthesis can also be a variable. Programmers commonly use a For Loop to count through all sections of an array and load data cells in the spreadsheet into the array. You’ll see how to do this later in this article.
How to Program a VBA Array in Excel
Let’s take a look at a simple program where you may want to load information from a spreadsheet into a multidimensional array.
As an example, let’s look at a product sales spreadsheet, where you want to pull the sales rep’s name, item, and total sale out of the spreadsheet.
Note that in VBA when you reference rows or columns, you count rows and columns starting from the top left at 1. So the rep column is 3, the item column is 4, and the total column is 7.
To load these three columns over all 11 rows, you’ll need to write the following script.
Dim arrMyArray(1 To 11, 1 To 3) As String
Dim i As Integer, j As Integer
For i = 2 To 12
For j = 1 To 3
arrMyArray(i-1, j) = Cells(i, j).Value
Next j
Next i
You’ll notice that in order to skip the header row, the row number in the first For look needs to start at 2 rather than 1. This means you need to subtract 1 for the array row value when you’re loading the cell value into the array using Cells(i, j).Value.
Where to Insert Your VBA Array Script
To place program a VBA script in Excel, you need to use the VBA editor. You can access this by selecting the Developer menu and selecting View Code in the Controls section of the ribbon.
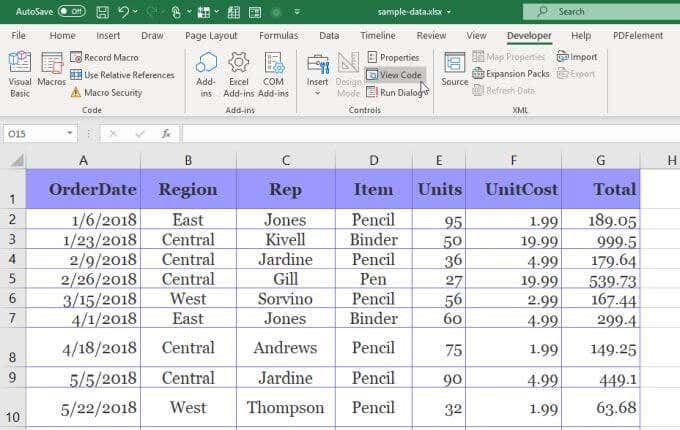
If you don’t see the Developer in the menu, you’ll need to add it. To do this, select File and Options to open the Excel Options window.

Change the choose commands from dropdown to All Commands. Select Developer from the left menu and select the Add button to move it to the pane on the right. Select the checkbox to enable it and select OK to finish.
When the Code Editor window opens, make sure the sheet your data is in is selected in the left pane. Select Worksheet in the left dropdown and Activate in the right. This will create a new subroutine called Worksheet_Activate().
This function will run whenever the spreadsheet file is opened. Paste the code into the script pane inside this subroutine.

This script will work through the 12 rows and will load the rep name from column 3, the item from column 4, and the total sale from column 7.
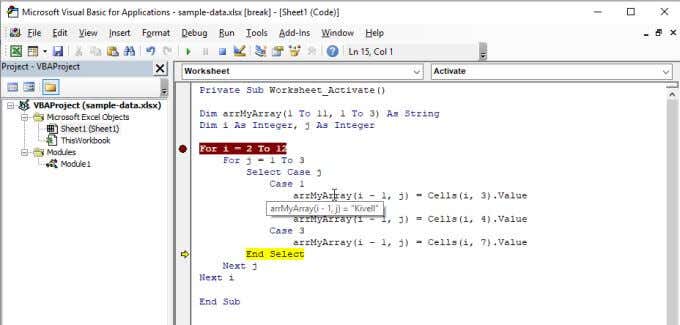
Once both For loops are finished, the two dimensional array arrMyArray contains all of the data you specified from the original sheet.
Manipulating Arrays in Excel VBA
Let’s say you want to apply a 5% sales tax to all of the final sale prices, and then write all of the data to a new sheet.
You can do this by adding another For loop after the first, with a command to write the results to a new sheet.
For k = 2 To 12
Sheets("Sheet2").Cells(k, 1).Value = arrMyArray(k - 1, 1)
Sheets("Sheet2").Cells(k, 2).Value = arrMyArray(k - 1, 2)
Sheets("Sheet2").Cells(k, 3).Value = arrMyArray(k - 1, 3)
Sheets("Sheet2").Cells(k, 4).Value = arrMyArray(k - 1, 3) * 0.05
Next k
This will “unload” the entire array into Sheet2, with an additional row containing the total multiplied by 5% for the tax amount.
The resulting sheet will look like this.

As you can see, VBA arrays in Excel are extremely useful and as versatile as any other Excel trick.
The example above is a very simple use for an array. You can create much larger arrays and perform sorting, averaging, or many other functions against the data you store in them.
If you want to get real creative, you could even create two arrays containing a range of cells from two different sheets, and perform calculations between elements of each array.
The applications are limited only by your imagination.